Extracting barcode information from PDF documents
Introduction
In this tutorial, we will look into how to use PassportPDF to extract information embedded in a barcode.
You should have your machine already set up using instructions from our getting started guide.
Extracting barcode information from PDF documents
In the following tutorial, we are going to:
- Load a document from a URI using DocumentLoadFromURIAsync.
- Extract barcode information using ImageReadBarcodesAsync or ReadBarcodesAsync depending on the document format.
The document we passed to the API looks like this:
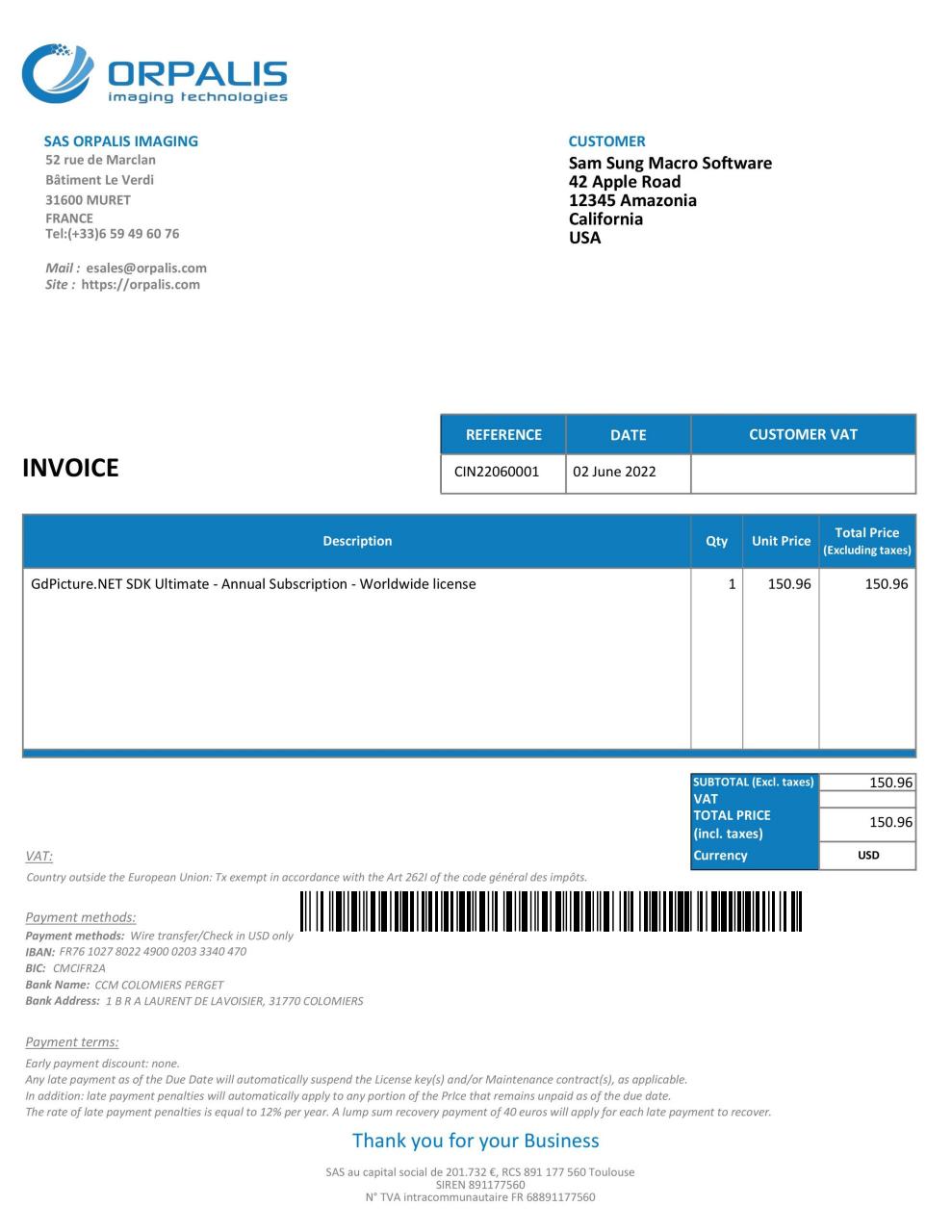
As you can see, there is a barcode on the invoice. It contains a message that we would like to extract. The code we used to extract this information is pretty simple, as shown below:
using PassportPDF.Api;
using PassportPDF.Client;
namespace BarcodeExtraction
{
public class BarcodeExtractor
{
static void displayBarcodesInfo(PassportPDF.Model.ReadBarcodesResponse barcodes)
{
foreach (var barcode in barcodes.Barcodes)
{
Console.WriteLine("Barcode type : {0}", barcode.Type);
Console.WriteLine("Barcode symbology : {0}", barcode.Barcode1DSymbology);
Console.WriteLine("X_left : {0}", barcode.X1);
Console.WriteLine("Y_top : {0}", barcode.Y1);
Console.WriteLine("X_right : {0}", barcode.X3);
Console.WriteLine("Y_down : {0}", barcode.Y3);
Console.WriteLine("Barcode data : {0}", barcode.Data);
Console.WriteLine("-------------------");
}
}
static void Main(string[] args)
{
GlobalConfiguration.ApiKey = "YOUR-PASSPORT-CODE";
DocumentApi api = new();
var uri = "https://passportpdfapi.com/test/invoice_with_barcode.pdf";
var document = api.DocumentLoadFromURIAsync(new PassportPDF.Model.LoadDocumentFromURIParameters(uri)).Result;
var imageSupportedFormats = new ImageApi().ImageGetSupportedFileExtensionsAsync().Result;
if (imageSupportedFormats.Value.Contains(document.DocumentFormat.ToString()))
{
ImageApi imageApi = new();
var barcodes = imageApi.ImageReadBarcodesAsync(new PassportPDF.Model.ImageReadBarcodesParameters(document.FileId, "*")).Result;
displayBarcodesInfo(barcodes);
}
else if (document.DocumentFormat == PassportPDF.Model.DocumentFormat.PDF)
{
PDFApi pdfApi = new();
var barcodes = pdfApi.ReadBarcodesAsync(new PassportPDF.Model.PdfReadBarcodesParameters(document.FileId, "*")).Result;
displayBarcodesInfo(barcodes);
}
else
{
var pdfImportSupportedFormats = new PDFApi().GetPDFImportSupportedFileExtensionsAsync().Result;
if (pdfImportSupportedFormats.Value.Contains(document.DocumentFormat.ToString()))
{
PDFApi pdfApi = new PDFApi();
var convertedDocument = pdfApi.LoadDocumentAsPDFFromHTTPAsync(new PassportPDF.Model.PdfLoadDocumentFromHTTPParameters(uri)).Result;
if(convertedDocument != null)
{
var barcodes = pdfApi.ReadBarcodesAsync(new PassportPDF.Model.PdfReadBarcodesParameters(convertedDocument.FileId, "*")).Result;
displayBarcodesInfo(barcodes);
}
}
else
{
Console.WriteLine("Document format not supported!");
}
}
}
}
}
For a full version of this code with all dependencies included, check out the complete project.
Now, let’s take a look at the response we received.
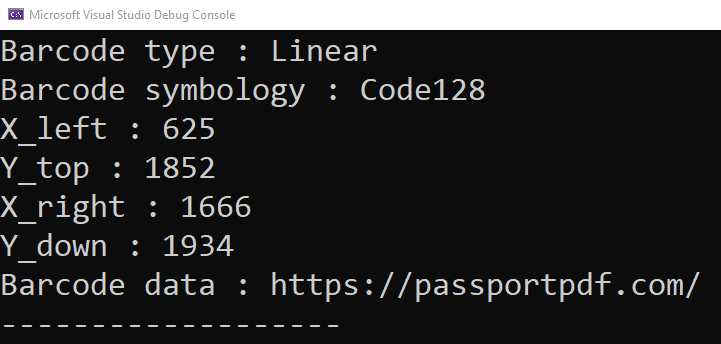
As you can see, we get a lot of information about the barcode, such as the type of barcode, its symbology, the coordinates, and the message or data embedded. In our case, the data is https://passportpdf.com/.
Final remarks
Using the barcodes reader from PassportPDF, you can read all sorts of barcodes, including:
- Linear barcodes.
- QR codes.
- Micro QR codes.
- Data matrix.
- PDF417.
- Aztec.
- Maxi code.
Also, PassportPDF supports many document formats such as JPEG, PNG, and a hundred more. So you can run the API on most documents that you have.